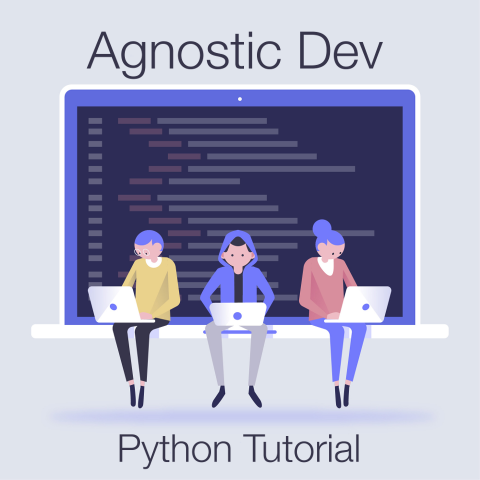
If you have ever wondered how to find a substring inside another string in Python then hopefully this tutorial will help walk you through how to do that. As an example, in Python 2.7 or above, if you have ever wondered how to determine if the substring "Agnosticdev," is part of the parent string "Hello Agnosticdev, I love Tutorials," then this tutorial will show you how to indicate, with a boolean value, the existence of the substring. This tutorial takes advantage of Python's Standard Library API methods that are most commonly used by Python developers for their day to day development operations. The Python Standard Library APIs contain a lot of useful information when working with string operations and before starting this tutorial it would be helpful to brush up on the difference between Python 2.7 and Python 3.6 before reading further.
NOTE: This tutorial assumes that you are at least using Python 2.7 or greater. The code samples in this tutorial will cover Python 2.7.13 and Python 3.6.1 and will be tested on Ubuntu (16.04) Linux and macOS Sierra 10.12.4. I have not tested the code in a Window's environment, but if you are using a Linux subsystem on Windows with Python available, the results should be fairly similar, but cannot be guaranteed.
Code Example:

The most straightforward and widely available example of how to determine if a substring is available in a parent string is by performing a boolean check to determine if a parent string is containing a substring value. This boolean check will return true if the substring is found and false if the substring is not found. Allowing your program to act upon that result accordingly. This code example is available for use in Python 2.7 and Python 3.6 and is a very low-risk and computationally moderate way for checking the existence of a substring in Python. If you can, I would take this approach in your program for sake of computation and simplicity.
string = "Hello Agnosticdev, I love Tutorials" substring = "Agnosticdev" # Straight forward approach for Python 2.7 and Python 3.6 # Executes the conditional statement when the substring is found if substring in string: print ("Your substring was found!")
Alternative Approaches:
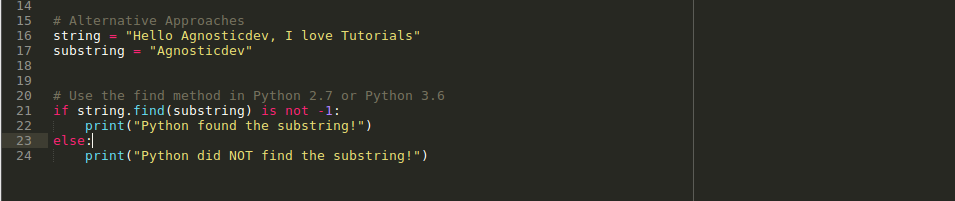
A very nice alternative approach to performing the if substring boolean check is to use the Python Standard Library string method for find(). This approach works very nicely because it is supported by Python 2.7 and Python 3.6. The idea behind this approach is to determine the starting index of the substring you are looking for. If you notice, I am performing a conditional check to determine if the result of the find method call is -1 or not. If the substring is not found, the find method will return -1. Meaning that the substring was not found in the parent string. If the substring is found, the find method will return the starting index of the first character of the substring. In this case the starting index is 6 because the first character of the substring is found at character 6 of the parent string.
# Alternative Approaches string = "Hello Agnosticdev, I love Tutorials" substring = "Agnosticdev" # Use the find method in Python 2.7 or Python 3.6 if string.find(substring) is not -1: print("Python found the substring!") else: print("Python did NOT find the substring!")
Now you know more about how to find substrings in parent strings in Python! Please feel free to download the Python source example from my Github and mess around with it. As always, if you have a comment or questions please feel free to reach out to me or leave a comment.
Thank you very much for reading!
Credits: Cover image designed by Freepik.
Comments
how to color string of characters in Text tkinter python 3.6
Hi
I'm looking for how to color a string of characetes in a Text widgte tkinter (python 3.6). at HTML,
we can use span tag :(I did not intentionally put < at the code below)
span class="mycolor" Hello, freinds your are welcome /span
style>
.mycolor {color: # FF0000; }
/style>
this sentence "Hello, freinds your are welcome" is written at each line with the color (# FF0000)
I want to use like this procedure in python 3.6 with string of characters
Hello Hamada,…
Hello Hamada,
Working with tkinter is a bit outside the scope of this article. I will point you towards the tkinter documentation though as what I understand you to be doing looks possible. https://docs.python.org/3/library/tk.html
.find method
This .find() cant return the number of occurance of a string.
If we use .count() then it returns how many time a string is present.
But that also fail to return value if string in below case
String = ABCDCDC
SUBSTRING = CDC
Anurag, thank you very much…
Anurag, thank you very much for the feedback. I will have a look into this.
Possible Solution
def count_substring(string, sub_string):
count = 0
for i, c in enumerate(string):
if sub_string == string[i:i+len(sub_string)]:
count += 1
return count
thank you
these piece of code helped me a lot
No problem, always glad to…
No problem, always glad to help.
Get index
How to get index of the last character of the string that is matched?