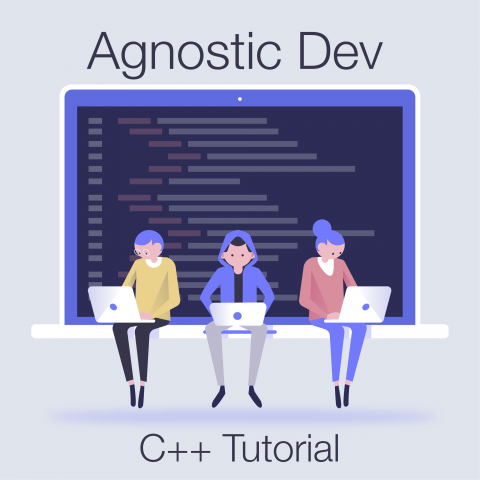
If you have ever wondered how to find a substring inside another string in C++ then hopefully this tutorial will help walk you through how to do that. As an example, in C++, if you have ever wondered how to determine if the substring "Agnosticdev," is part of the parent string "Hello Agnosticdev, I love Tutorials," then this tutorial will show you how to indicate, with an unsigned integer, the index of the first character in the substring. This tutorial takes advantage of C++ Standard Library String functions that are most commonly used by C++ developers for their day to day development operations. The C++ Standard Library contain a lot of useful information when working with string functions and before starting this tutorial it would be helpful to brush up on some of the basic syntax here.
NOTE: This tutorial is compiled using clang on macOS (Apple LLVM version 8.1.0 (clang-802.0.42)). However, you will probably see that my code example is using gcc to compile C++. I do not actually have gcc installed on my system and Apple has linked all commands from gcc to clang for back support. The code in the example has not been tested on Linux or Windows but there is no reason this code should not also run in an environment with gcc present like Linux or a Windows/Linux subsystem.
Code Example:

In this first example we declare a string and then use the string standard library find function to get the position of the first character of the substring in the parent string. This is the most straight forward way to find a substring and I would advise this approach if you are using a standard library string.
#include <iostream> #include <string> /* Apple LLVM version 8.1.0 (clang-802.0.42) References: find : http://www.cplusplus.com/reference/string/string/find/ length : http://www.cplusplus.com/reference/string/string/length/ Compile: g++ -o substring substring.cpp */ int main () { std::string parentstring = "Hello Agnosticdev, I love Tutorials"; std::string substring = "Agnosticdev"; if (std::size_t index = parentstring.find(substring)) { std::cout << "Substring found at index " << index << ", with length " << substring.length() << std::endl; } else { std::cout << "Substring not found at all in parent string" << std::endl; } return 0; }
*** EDIT ***
A good point that was brought up to me on this tutorial is that if you search for the word "Hello" in the parentstring then the index of 0 is returned. If 0 is returned then the if statement would fail and the previous method I had described above would only be usable for substrings that are found at an index greater than 1. An alternative approach would be to check for the second character of the substring starting at index 1. In the example below the zeroIndexChar would return as 1 and the substring would be found at 0.
std::string parentstring = "Hello Agnosticdev, I love Tutorials"; std::string availableSubstring = "Agnosticdev"; std::string substring = "Hello"; std::string zeroIndexChar = "ello"; if (std::size_t index = parentstring.find(substring) && index != 0) { std::cout << "Substring found at index " << index << ", with length " << substring.length() << std::endl; } else if (parentstring.find(substring) == 0 && parentstring.find(zeroIndexChar) == 1) { std::cout << "Substring found at index 0, with length " << substring.length() << std::endl; } else { std::cout << "Substring not found at all in parent string" << std::endl; }
Alternative Approaches:
An alternative approach than using the find public member function is to build a routine that captures the first index of the first substring character without the find method. In this alternative approach a pointer is created to the parent string and then a pointer to a c-string array of characters is created with the original character used in the parent string. The parent string array of characters is then looped through and at each iteration a conditional check is made to determine if the current parent string character is equal to the first character in the substring. If there is a substring character match then the index position is saved and the comparison continues character by character to determine if each character is a match, all the way to the end of the substring. If the substring is completely matched then the first character position is returned.
#include <iostream> #include <string> /* Apple LLVM version 8.1.0 (clang-802.0.42) References: c_str : http://www.cplusplus.com/reference/string/string/c_str/ length : http://www.cplusplus.com/reference/string/string/length/ Compile: g++ -o substring substring.cpp */ int FindSubstringindex(const std::string *parentstring, const std::string *substring) { int e = 0, indx = -1; const char *parentcstring = parentstring->c_str(); const char *csubstring = substring->c_str(); for (int i = 0; i < strlen(parentcstring); i++) { if (parentcstring[i] == csubstring[e]) { if (e == 0) indx = i; e++; if (e == strlen(csubstring)) { return indx; } else { e = 0; } } } return -1; } int main () { const std::string extendedParentstring = "Hello extended string Agnosticdev, I love Tutorials"; const std::string substring = "Agnosticdev"; // Alternative Approach int substringIndex = FindSubstringindex(&extendedParentstring, &substring); std::cout << "Substring found at index " << substringIndex << std::endl; return 0; }
Now you know more about how to find substrings in parent strings in C++! As always, if you have a comment or questions please feel free to reach out to me or leave a comment. You can download the code used in this tutorial on my GitHub here.
Thank you very much for reading!
Credits: Cover image designed by Freepik.
Comments
a typo in FindSubstringindex ?
Looks like there is no ending bracket in FindSubstringindex for 'for' or 'if'
Thank you very much, John. …
Thank you very much, John. I made the correction on the ending bracket on the if statement. Much appreciated.
std::string::find returns…
std::string::find returns npos constant if not found, which is max assignable value of size_t. So testing for "truthiness" of return value is wrong, and the complicated stuff is not actually needed.
Roman, thank you for your…
Roman, thank you for your feedback. Yes, you are right. std::string::find returns npos (Maximum value for size_t) if nothing is found. In the example above I wanted to make sure that the user did not fall into a failing if statement though if the substring was found as index 0. This is the only reason for verifying the return values. Another option I could have illustrated is to perform the find call outside of the if statement.
Thank you!
Suggestion
int main () {
std::string parentstring = "Hello Agnosticdev, I love Tutorials";
std::string substring = "Agnosticdev";
if ((std::size_t index = parentstring.find(substring)) && index != std::string::npos) {
std::cout << "Substring found at index " << index << ", with length " << substring.length() << std::endl;
} else {
std::cout << "Substring not found at all in parent string" << std::endl;
}
return 0;
}
Thank you very Suggestor,…
Thank you very Suggestor, std::string::npos is also another nice way to make sure that the index is found and is not negative.
Appreciate the feedback.
std::string::npos reference: http://www.cplusplus.com/reference/string/string/npos/
findsubstringindex does not work
The variable e is incremented and then is set to zero. Then it looks again.