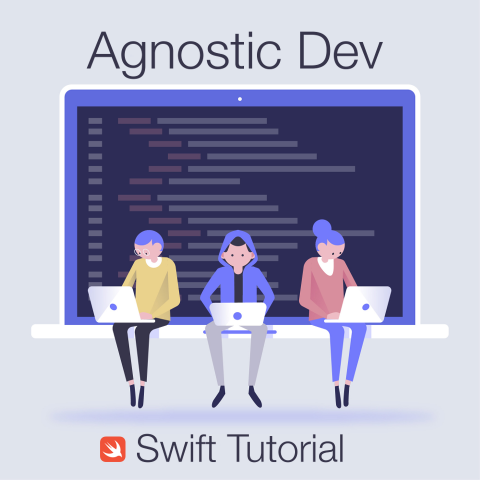
If you are like me and sometimes you forget the syntax on how to sort an object by a custom property then this tutorial is aimed at you. The goal for this tutorial is to show you how to sort custom objects in Swift by a date property. But that's not it! One of the major reasons why the API used in this tutorial is so versatile is because it can also be extended out to other properties on a custom object like a string property, for example. So by the end of this tutorial you should be able to sort collections of objects by either date or string properties in either Swift 3 or 4.
NOTE: This tutorial was created using Xcode 9.2 and assumes you have at least Swift 4.0 installed in your Xcode toolchain or that you are using a version of Xcode that supports Swift 4, like Xcode 9. The API used in this tutorial is supported in Swift 3/Xcode 8 though. To get started with this tutorial you can know a lot about Swift or a little about Swift, these examples are aimed at everyone. 👍
Setup the Basics 👨💻
In the code example below I am doing a bit of setup work to illustrate the example I am about to demonstrate. First, I create a custom class with a three properties; title, desc, and date. These properties are set when the object is created by the values passed into the required constructor. The date property is assigned a date format according to the format that is used from my blog. From here, the date is formatted and the date is correctly created from the passed in string and the date is set to the local date property. Next, five objects are created with random dates and titles to showcase sample data created with different dates and times. Lastly, the dates and titles are printed out to illustrate that the objects are not in any sort of order.
class customObject: NSObject { var title: String = "" var desc: String = "" var date: Date = Date() required init(title: String, desc: String, dateString: String) { // Set the title and description self.title = title self.desc = desc // Set the date formatter and optionally set the formatted date from string let dateFormatter = DateFormatter() dateFormatter.dateFormat = "MMMM d, yyyy" if let date = dateFormatter.date(from: dateString) { self.date = date } } } // Create 5 sample objects for testing, all with sporadic dates var obj3 = customObject(title: "TLS 1.3 - Better, Stronger, Faster", desc: "Overview of TLS 1.3", dateString: "January 6, 2018") var obj4 = customObject(title: "User Interface Testing with Swift and XCTest", desc: "Overview of UI Testing", dateString: "December 10, 2017") var obj2 = customObject(title: "How to Use Python List Comprehensions", desc: "Overview of Python List Comprehensions", dateString: "December 2, 2017") var obj1 = customObject(title: "Attending WWDC 2017 - Predictions Answered", desc: "Predictions Answered on WWDC 2017", dateString: "June 13, 2017") var obj5 = customObject(title: "Swift Network Testing - Automate XCTest with Python", desc: "Automate XCTest with Python", dateString: "November 26, 2017") // Display the dates and titles print("Unsorted Date from obj1: \(obj1.date) with title: \(obj1.title)") print("Unsorted Date from obj2: \(obj2.date) with title: \(obj2.title)") print("Unsorted Date from obj3: \(obj3.date) with title: \(obj3.title)") print("Unsorted Date from obj4: \(obj4.date) with title: \(obj4.title)") print("Unsorted Date from obj5: \(obj5.date) with title: \(obj5.title)\")
Sorting the Objects 🎉
Here is where the magic happens. Below we assemble all of the newly created objects in an array called customObjects. Next, the sorted(by:) API is used to sort the collection and compare each object in the collect by the given property of the object. In this case we are sorting by the .date property and we are arranging the objects to be in descending order using the .orderedDescending case.
After the customObjects array is sorted, each object is then printed out using a for loop to display the finished result!
// Now, with 3 short lines of code is where the magic happens // First the objects are wrapped up in a generic array var customObjects = [obj1, obj2, obj3, obj4, obj5] // Next, the .sorted(by:) method returns a collection that compares an element in the array against the next element and arranges the collection by date. // The sorted collection is assigned back to the customObjects array for display customObjects = customObjects.sorted(by: { $0.date.compare($1.date) == .orderedDescending }) // The sorted customObjects collection is then printed out to display the objects sorted descending by date for obj in customObjects { print("Sorted Date: \(obj.date) with title: \(obj.title)") }
In Summary ⌛️
Now you know more about how to sort objects by date and string in Swift 3 or 4! Please feel free to download the playground example from my Github and mess around with it. One thing to note again is that this tutorial was created using an Xcode toolchain supports Swift 4, but the API used in this tutorial supports Swift 3. Please download Xcode 9 before you run the playground from the link above.
Thank you very much for reading!
Credits: Cover image designed by Freepik.
Comments
Swift
Comparing any 2 object types that are not the same will result in a casting exercise and several lines of code, all of which could have been achieved in Objective C with a single line of code.
Swift
What if you wanted to Sort objects by date anytime after the current date?