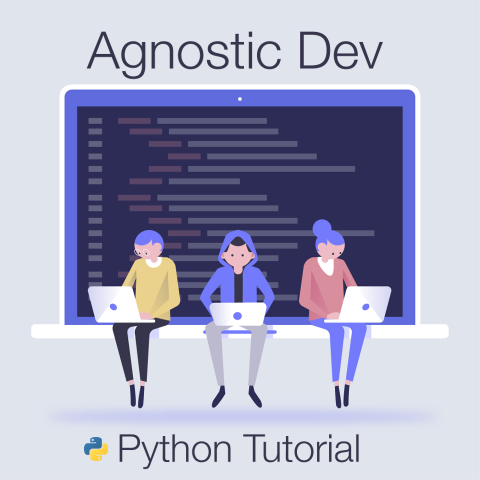
If you have ever wanted to use Python list comprehensions, but were unsure of the syntax or exactly how they worked, then this tutorial is aimed at you. This tutorial aims to walk you through the basics of how Python list comprehensions work with three real world examples. In the tutorial below I have three examples of the power of Python list comprehensions; The first example illustrates how to setup and create a basic list comprehension. The second example get's a lot more complex and uses two nested for loops with a added list to create a two-dimensional matrix. The third example uses an optional conditional statement in the list comprehension to check for even or odd numbers.
🐍 Python list comprehensions are a great utility and can greatly reduce the amount of code that is used to get a job done. Before we begin with examples though, I thought it would be good to mention the different the parts to a Python list comprehension. I look at these parts as the building blocks of a Python list comprehension, and they come in three different parts. First is the output, which is the statement evaluated to provide the output that is used from the statement. Next is the for loop(s) that run to compute to give the output values to compute. Last is an optional conditional statement. This conditional statement can be used to make sure values are evaluated before they are given to the output.
[ (output) (for loop(s)) (condition) ]
NOTE: This tutorial assumes that you are at least using Python 2.7 or greater. The code samples in this tutorial will cover Python 2.7.10 and Python 3.6.1 and will be tested on Ubuntu (16.04) Linux and macOS High Sierra 10.13.2. I have not tested the code in a Window's environment, but if you are using a Linux subsystem on Windows with Python available, the results should be fairly similar, but cannot be guaranteed.
🐍 Basic Example:
The following example will create a list of squared numbers out of an existing list.
l = [4, 5, 6, 7, 8] squared_list = [] for n in l: squared_list.append(n*n) # Output is 16, 25, 36, 49, 64
Now, let's take a look at how to perform the same routine, but this time using a Python list comprehension. In our list comprehension example the list that contains the numbers (l) is looped through and the output statement does the work of squaring the number to be added to the squares_list for usage.
squared_list = [n * n for n in l] # Output is 16, 25, 36, 49, 64
🐍 2D Matrix Example:
The following example will loop through the dx values and multiply each one by a dy value and add this value to a row. This matrix takes the shape of a NumPy matrix.
dx = [3, 4, 5, 9] dy = [5, 3, 2, 1] output_matrix = [] for dx_n in dx: dx_row = [] for dy_n in dy: dx_row.append(dx_n * dy_n) output_matrix.append(dx_row) # Output is [[15, 9, 6, 3], [20, 12, 8, 4], [25, 15, 10, 5], [45, 27, 18, 9]]
Now, let's take a look at how to perform the same routine, but this time using a Python list comprehension. In our list comprehension example the output value is again multiplied but now you will see that I have two different for loops running in my comprehension; one for dy and one for dx. The loops are running backwards so that the output is evaluated the same way a conventional nested set of loops would evaluate the data.
output = [[dx_n * dy_n for dy_n in dy] for dx_n in dx] # Output is [[15, 9, 6, 3], [20, 12, 8, 4], [25, 15, 10, 5], [45, 27, 18, 9]]
🐍 Conditional Evaluation Example:
The following example will iterate through a list of numbers and evaluate the even numbers to be added to a final list for usage.
l = [3, 4, 5, 6, 8, 9, 11, 12, 13, 14, 15] even_list = [] for num in l: if num % 2 == 0: even_list.append(num) # Output is 4, 6, 8, 12, 14
Now, let's take a look at how to perform the same routine, but this time using a Python list comprehension. In our list comprehension example we are using a single for loop once again, but this time on each iteration of the loop we are checking if num can be used in the output based upon the output of the conditional step. The conditional step check for the num value to be either even or odd and if the value is even the num value is added for usage in the output step.
even = [num for num in l if num % 2 == 0] # Output is 4, 6, 8, 12, 14
In Summary ⌛️
Where to go next? You can find all of the code from this post up on my Github Repo here if you want to take a look of try it out on your own. Please let me know if you have any questions, comments or concerns on any of the examples I ran through. As always, thank you for reading!
Credits: Cover image designed by Freepik.
Comments
Python Codings
Above given Matrix examples are very useful to me. I want to know how loop are run and there types. Above info was very useful to me.
Thank you
Very good explanation.Thank you
No problem, thank's for the feedback!
Thank's for the feedback, Subham. I am glad you found the tutorial informative.
Informative
Very nice explanation, thank you