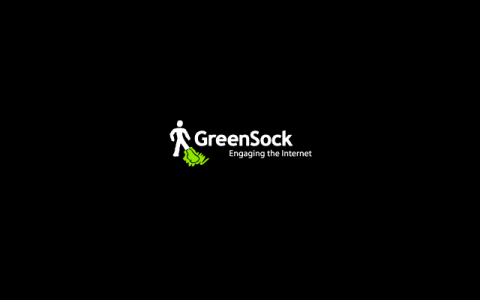
Web animation just became a whole lot easier using the GreenSock Animation Platform. The GreenSock JavaScript libraries give you a vast array of options when selecting the necessary tools to make any animation on your site come alive.
In this tutorial, I’m going to demonstrate how to animate a series of potential background images using the GreenSock library TimelineMax.
TimelineMax lets you setup a series of animation events called “Tweens” that act as a waypoint in your timeline to execute a designed animation at a certain time or label. Lets jump right in.
First we declare the TimelineMax object. Notice that the object is set to “paused,” this is so the timeline does not immediately execute once the tweens are added to it.
var tl = new TimelineMax({paused:true});
Next we declare all of our variables needed in setting up the UI and an array of labels to be used in our timeline.
Notice that we are using jQuery here also. GreenSock and jQuery work very well together which is another added bonus to working with GreenSock.
var labels = ["label-one", "label-two", "label-three"]; var $window = $(window), index = 0; var h = $window.height(), w = $window.width(); var $img = $('.image img'); var $con = $('.image'); var $container = $('#container'); var $up = $('#up'), $down = $('#down');
Next I am adding all the URLs to the images in the DOM and setting the height and width of the image container elements.
var img_array = [ "http://www.lorempixum.com/"+ w +"/"+ h +"/city", "http://www.lorempixum.com/g/"+ w +"/"+ h, "http://www.lorempixum.com/"+ w +"/"+ h +"/sports", "http://www.lorempixum.com/"+ w +"/"+ h +"/abstract"]; $con.css({'width':w, 'height': h}); var e = 0; $.each($img, function(index, value){ $(value).attr('src', img_array[e]); e++; });
Here is where we build our GreenSock timeline. Each animation is defined with an element, a time value for the duration of the animation, a css property, an easing equation, and a label to identify the each tween in the timeline.
The css property is actually what is making the $container element move, this is the actual value that GreenSock is animating.
// element time css property easing equation label tl.to($container, 0.8, {css:{top:-(h)}, ease:Power4.easeOut}, labels[0]); tl.to($container, 0.8, {css:{top:-(h * 2)}, ease:Power4.easeOut}, labels[1]); tl.to($container, 0.8, {css:{top:-(h * 3)}, ease:Power4.easeOut}, labels[2]);
Here we setup event handlers for each of the buttons that appear at the top and bottom of the screen.
If the user presses the “UP” button then the timeline will move forward and animation to the next label on the screen using the TimelineMax method, tweenTo(). If the user presses the “DOWN” bottom then the timeline will animate backwards using the tweenTo() method also.
//our jQuery click events $up.on('click', tweenDown); $down.on('click', tweenUp); //our tween up function to move the timeline forward function tweenUp(){ if(index == 3) index = 3; else index++; //here we tween forward to the designated label //think of this as a waypoint or keyframe to send our animation to tl.tweenTo(labels[index]); //hide or show our buttons using jQuery if(index == 3){ $down.css('display', 'none'); $up.css('display', 'block'); } } //our tween down function to move the timeline backward function tweenDown(){ if(index == 0) index = 0; else index--; //here we tween backward to the designated label on our timeline //think of this as a waypoint or keyframe to send our animation to tl.tweenTo(labels[index]); //hide or show our buttons using jQuery if(index == 0){ $down.css('display', 'block'); $up.css('display', 'none'); } }
Here’s the HTML
<div id="wrapper"> <div id="container"> <div class="image one"><img src="" alt="Pic 1" /></div> <div class="image two"><img src="" alt="Pic 2" /></div> <div class="image three"><img src="" alt="Pic 3" /></div> <div class="image four"><img src="" alt="Pic 4" /></div> </div> <div id="down"><div class="text">DOWN</div></div> <div id="up"><div class="text">UP</div></div> </div>
Here’s the CSS
body{ background-color:#555; padding:0; margin:0; font-family:Arial, Helvetica, sans-serif; height: 100%; overflow: hidden; } #wrapper{ position: relative; width: 100%; height: 100%; } #container{ position:absolute; width:100%; } #container img{ width:100%; height:100%; } #down{ position: absolute; top:0; width:120px; height:40px; cursor:pointer; opacity:0.8; background-color:#222; color:#fff; z-index:100; float:right; right:50px; } #up .text{ margin:10px 0 0 48px; } #down .text{ margin:10px 0 0 34px; } #up{ position: absolute; bottom:0px; width:120px; height:40px; cursor:pointer; opacity:0.8; background-color:#222; color:#fff; z-index:100; float:right; right:50px; display:none; }
That’s it! That was pretty awesome, was it not? Check out all GreenSock has to offer with both their JavaScript libraries and their ActionScript libraries here: http://www.greensock.com/ . You are welcome to download the files or view the demo below for further details.
If anyone has any questions or concerns please post your responses, I’d love to hear from you
Download Tutorial Files Below.
Comments
WONDER OF SCIENCE
I love your well-written Post,your article help me to get some ideas.
Thanks for you Share This Amazing Post,
ios 7 animations, ios SDK
WRAPPER
wrong CSS "#wraper" missing a "p"
CSS SELECTOR
@RawBird, Thank you. I've updated my post.