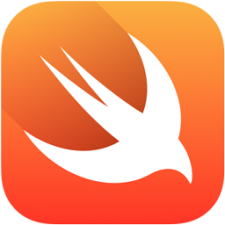
This Monday in San Francisco Apple had their annual World Wide Developer Conference or WWDC. Last year Apple debuted iOS7, and it was a significant release for designers. This year, Apple announced iOS8, and they promised it to be a “Giant” developer release, a release that has not been this large since the launch of the app store.
Some of the new developer releases that were discussed at WWDC this year were Extensibility, HomeKit, HealthKit, Metal, and SpriteKit, but the one I thought to be the most interesting and it seems to be the one getting the least amount of attention is Swift, the new Apple Developer language. Swift is promised to be Objective-c, but without the baggage of C. Sounds kind of weird, right? After all, is not the iOS platform become what it is today because of the power of C? I did not want to be too judgmental and I also did not want to just take Apple's word for it, so I thought I would do my own research into how the new Swift programming language really works. Here is what I found:
Swift can be written side by side in an application with Objective-C, but it cannot be written in the same file. Swift uses the .swift file extension and of course Objective-C uses the .h and .m file extensions. Swift can be written in Xcode or in Playground. Playground is essentially Xcode, but is available as a real time execution window that can test snippets of Swift code as you write them without having to run them in Xcode, or creating testing projects to test out segments of code. Playground uses the .playground file extension. Writing Swift code at first seemed like writing a preprocessor for Objective-c, but once I dug deeper into the language it seems more like writing a scripting language, like Python or JavaScript. This is interesting because I wonder if this was the intention of the creators of Swift. Scripting languages are extremely popular because of the low barrier for entry and the instant results that it gives a developer, you have to wonder if Apple is trying to attack a wider range of developers by making iOS development seem like developing Python, Lua, or JavaScript.
Taking a look at the actual syntax differences between Objective-C and Swift I found many interesting features that were not possible in Objective-C that are now offered in Swift. To help illustrate some of those differences I provided some examples below.
Variable declarations seem to be a lot less code than Objective-c and very similar to Python. For example, say I want to create an array with three strings in it.
//Objective-C : NSArray *arr = [NSArray arrayWithObjects:@"stringOne", @"stringTwo", @"stringThree", nil]; //or NSArray *arr = @[@"stringOne", @"stringTwo", @"stringThree"]; //Swift: var arr = ["stringOne", "stringTwo", "stringThree"]
Say for example, you would like to add another dynamic set of data in the middle of a string.
//Objective-C NSString *adjective = @"cool"; NSString *dynamic = [NSString stringWithFormat@"My sentence is really %@", adjective]; //Swift let adjective = "cool" let dynamic = " My sentence is really \(adjective)"
Another interesting feature about Swift compared to Objective-C is that in Swift you have the option to explicitly to declare a data type if you wis or have Swift choose one for you based upon the data that you assign to a variable. For example, consider the following:
//Swift //the following data type would be considered an integer because you are assigning a non-floating point //value let myVariable = 33 //the following data type would be considered a Double because of the decimal value that is assigned let myVariable = 3.4454 //you can also write it with a semi colon, Swift does not care let myVariable = 3.4454;
Working with functions is a lot more simplified too with some very interesting and powerful new features that are available in Swift that were not available in Objective-C. For example, in Swift, you can nest functions and also pass a function call as an argument in a different function call.
For example, lets say I wanted to take two functions working together to perform a task, in Objective-c, I would need to create two different functions, but in Swift all that functionality can live inside the parent function and the child function can inherit scope over the arguments passed into the parent function. To demonstrate this new feature, here is an example of generating random even numbers that fall in between a certain range. Obviously, this functionality does not require two functions to get the job done, but what is does is show that in Swift, the high and the low integers used in the generateRandom() function inherit the values passed into the parent function of returnEvenRandom().
//Objective-C -(int)returnEvenRandom:(int)low andHigh:(int)high { int num = [self generateRandom:low andHigh:high]; if((num % 2) != 0){ return ++num; } return num; } -(int)generateRandom:(int)low andHigh:(int)high { return low + (int)arc4random_uniform(UINT32_C(high - low + 1)); } NSLog(@"Random Even Number %d", [self returnEvenRandom:3 andHigh:24]); //Swift import Cocoa func returnEvenRandom(low: Int , high: Int) -> Int { func generateRandom() -> Int{ return low + Int(arc4random_uniform(UInt32(high - low + 1))) } var num = generateRandom() if(num % 2) != 0{ return ++num } return num } println(returnEvenRandom(3, 24))
Another very interesting new feature of Swift is returning tuples from a function. In Objective-C this is currently not possible unless you were to return and NSArray or NSDictionary holding the multiple values. This is a great feature, but will be restricted to use only with other swift code.
//Objective-C -(NSMutableArray *)returnMultiple:(int)one withTwo:(int)two { NSMutableArray *arr = [NSMutableArray array]; [arr addObject:[NSNumber numberWithInteger:one]]; [arr addObject:[NSNumber numberWithInteger:two]]; [arr addObject:[NSNumber numberWithInteger:(one + two)]]; return arr; } NSLog(@"Multiple %@", [self returnMultiple:4 withTwo:8]); //Swift func returnMultiple(one : Int, two : Int) -> (Int, Int, Int){ return (one, two, (one + two)) } println(returnMultiple(4, 8))
Working with classes and objects in Swift is pretty similar to working with classes and objects in Objective-C and C. Not much has changed, but the fact that you have full support to do so is great news if you are looking to completely make the switch to swift or if you are looking to use swift to represent your data models. As an example, here is a class developed in Swift that may be used to represent a user object in your application.
//Swift class user { var id : String; var name : String; var email : String; init(name: String, id : String, email : String){ self.name = name self.id = id self.email = email } func getDescription() -> String{ return "User name \(name) and email \(email) " } } var u = user(name: "Matt", id: "2s34ds", email: "agnosticdev@gmail.com") println(u.getDescription())
All in all I think Swift adds value to the iOS development platform by giving the developer options to either develop portions of the application in Swift or the whole application in Swift. I am going to start writing all of my utility and data manipulation classes in Swift as it proves to be valuable right away in those areas over Objective-C. Please tell me your thoughts or concerns about Swift. I would love to hear them.
Comments
IN MY OPINION, I SEE NOTHING
In my opinion, I see nothing valuable Swift brings comparing to Objective-C. Tuples? Maybe. But even in your example you can rewrite Objective-C code to use the literals:
-(NSArray*) returnMultiple: (int) one withTwo: (int) two {
return @[@(one), @(two), @(one+two)];
}
(Append 'mutableCopy' if you need to return NSMutableArray).
But there is a big drawback in Swift, comparing to Objective-C: it doesn't have macros.
I AGREE THAT TUPLES MAY BE A
I agree that Tuples may be a weak example for Swift when you can just return an NSArray in objective-c.