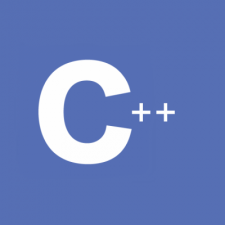
Lately I have been writing a lot of C++ on Linux and have been really enjoying it. I love the flexibility of jumping right in on the terminal, writing my code, and compiling all from one spot. As my writing continued and my program evolved I realized that I needed some worker threads to process some pretty expensive computation that I did not want hogging my main thread. Coming from an iOS/OSX background I figured I could do some research and figure out a comparable replacement for how I would normally create background threads with Grand Central Dispatch. I soon found out that there is not a one-for-one replacement for GCD when working with threading in C/C++. In fact, there is really nothing comparable in C/C++ to how iOS aggressively delegates background threads. Something like GCD would have to be implemented by hand through a custom signaling process to try and achieve asynchronous behavior. One of the better solutions I did find to my overall threading question was Pthreads or POSIX threading. Pthreads, although not as strong as a library like GCD, do work very nicely for processing tasks off of the main thread. Think of PThreads in you application as a split in processing power for computation to run in parallel for two different tasks.
So, what are are Pthreads anyways, and how are they used? Well, starting from the beginning PThreads are short for process threads and can be thought of as a independent tasks separate from the main task all managed and ran from a specific process in the operating system. All of the PThreads in a program can share the same memory and data, but to ensure thread safety all worker threads should maintain a scope of variables as to not cause collisions in memory. Each PThread can be locked using specific mutex locks on data that is global to the application, but in my opinion is is good to only maintain a local scope for what is needed in each PThread and let a PThread perform the computation that it needs to while the main thread runs all user interface interaction.
To illustrate these points I created a sample program below that uses thread safe data and global data to process information in the background and return it to the main thread. Let me know if you have any questions or I need to make some corrections.
/** * @file main.cpp * Demonstration program to illustration pthreads. **/ #include <iostream> #include <pthread.h> using namespace std; // Global Definitions struct thread_data { int threadData; }; /** * This is the computation that is running on the background thread **/ void * perform_computation(void * ptr) { struct thread_data *data = (thread_data *)ptr; data->threadData += 4; pthread_exit(0); } /** * The main function of this program **/ int main(int argc, const char * argv[]) { struct thread_data thrd; pthread_t thread_id; int mainData = 3; thrd.threadData = 3; thrd.threadData += mainData; std::cout << "Here is the thread data in the main " << thrd.threadData << std::endl; if (pthread_create(&thread_id, NULL, perform_computation, &thrd)) { std::cout << "Creating thread failed " << std::endl; return 0; } pthread_join(thread_id, NULL); std::cout << "Result of thread computation " << thrd.threadData << std::endl; return 0; }
To compile this program using g++ from the command line use the following commands:
$ g++ -pthread main.cpp -o pthreadProgram $ ./pthreadProgram Here is the thread data in the main 6 Result of thread computation 10
Comments
PTHREAD IS A REALLY NICE
Pthread is a really nice threading library but, since you're writing C++, you might want to have a look at std::thread, which provides a more platform-independent way for threading. Here's a refernce for it: http://en.cppreference.com/w/cpp/thread/thread
BTW, I like your writing style!
STD::THREAD
Kimmo Surakka, thank you very much for the feedback. I have received a lot of feedback about my decision to use Pthreads over std::thread, so I think I may investigate the same program as I used in this post but using std::thread instead and see how they match up.
WHY USE PTHREADS INSTEAD OF C
Why use pthreads instead of C++11/C++14's native threading?
Follow discussion on G+ https://plus.google.com/+MattEaton815/posts/GgLimWJRijj
I AM SORT OF A NEWBIE TO
I am sort of a newbie to threading in C++. I was unsuccessful getting native threads running in Ubuntu Linux so I turned to Pthreads.
IF YOU'RE A FAN OF
If you're a fan of libdispatch (GCD), you could use lib dispatch.
Follow discussion on G+ https://plus.google.com/+MattEaton815/posts/GgLimWJRijj
I JUST DISCOVERED LIBDISPATCH
I just discovered libdispatch. It looks like another interesting possibility, thanks!
Very interesting article
Perfectly written!
I will right away seize your rss feed as I can’t find your
e-mail subscription link or newsletter service.
Do you’ve any? Kindly let me understand in order that I could subscribe.