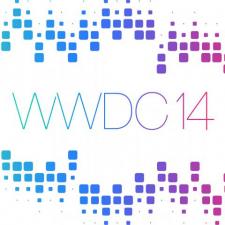
How and why Swift started at Apple is still a bit of a mystery to the outside world and has been the focal point of many rumors and speculation over the years. If I had to pick one place where there is solid evidence of Swift’s roots at Apple, it would have to be with Chris Lattner. Chris Lattner is the internal founder of the Swift language at Apple, with a claim from Lattner on his personal website that the language dates back to 2010 internally at Apple.
The origins on why the language started at Apple, is like I mentioned, still the mysterious part. If I had to sum all of the rumors and discussion up into one sentence, it would be that Swift was started out of a need to replace Objective-C. Objective-C has been the application language of choice to develop any macOS or iOS application in the Apple ecosystem for the last 21 years. And over time Objective-C has accumulated a lot disdain both internally at Apple and externally from outside application developers. Out of this disdain, and led by a core group at Apple, Chris Lattner developed the Swift language, but kept it a secret all the way up until 2014.
Apple developers fondly reminisce about where they were the first time they heard the news about the Swift language being released. I certainly remember where I was; I was sitting in the collaboration space at my old former employer watching the live stream of WWDC (World Wide Developers Conference), thinking to myself, “what just happened?” Objective-C, C, and C++ all interacting in one codebase has worked so well for such a long time. Why go and change a good thing? Turns out, I was not the only Apple developer that felt this way. For a good six months to even a year, I would say that the decision to release the Swift language divided the Apple community and drove some large companies to consider alternative approaches. For example, Facebook’s React Native emerged out of this announcement letting companies like Walmart, Facebook, Instagram, and Tesla not have to rebuild their application over in Swift.
Apple developers fondly reminisce about where they were the first time they heard the news about the Swift language being released.
After the initial shock of the Swift language announcement at Apple’s Worldwide Developer Conference, there was a lot of movement in the Swift language in that first year. A lot of problems with interoperability with Objective-C and Swift. And a lot of application binary interface stability issues with other programming languages interacting with Swift. Many Apple developers were turned off and decided to make other choices in the business of building applications.
From my own personal experience, I remember how rough of a start Swift had the first 12 months because I released an application with Swift built features in it in October of 2014. This was 4 months after Swift was released. When my application hit the iTunes store it crashed because of a dynamic library run-time issue on the device loading the application. Many other developers faced similar issues, but as the language started to evolve, rocky times were certainly ahead for Swift.
On December 3rd, 2015 Swift was released on Github as an official open source project under the Apache 2.0 license. This marked the dawn of a new era for Apple as a company that once kept everything close to the chest is now releasing their new development language on Github to be developed completed in the open. The move to Github for the Swift language was very exciting and very different as the Swift language is now in it’s second major release. I wish I could say that things were starting to become more stable for the language but the language was still undergoing a lot of transformation and experiencing a lot of stability issues. Over the last year and a half, since the release of Swift on Github, Swift has operated in the open with a evolution group called Swift Evolution. Swift Evolution is a group of standards writers that review and accept proposals for the Swift language. This has been a major step forward for the language because now Swift gets official input from community members.
Today we are on the doorstep of the release of Swift 4 to the masses.
Today we are on the doorstep of the release of Swift 4 to the masses. It is out in beta and the Swift core team is working on stabilizing the languages and providing ABI stabilization across all standard libraries. Swift 4 now is in a much much better place than it was in December of 2015 when it was released on Github. This fact along tells me that there is a benefit to developing a language out in the open. It tells me that the language has greatly benefited in a short amount of time from the evolution group and Apple’s willingness to take community input. I think that over the next couple of years Swift will continue to see a benefit from this and the language is only going to continue to grow stronger.
Swift as a Language (Some of the Key Features)
Swift as a language is actually very interesting and fun to write. I think the authors of Swift did a nice job in providing type safety in anyone making the transformation from Objective-C to Swift by allowing developers to take advantage of features familiar in Objective-C while writing Swift code. This common ground between languages allowed developers to learn Swift while utilizing their knowledge from Objective-C. This is certainly one ways I learned to write Swift code.
Swift from a language perspective is a compiled language, but can be thought of as a blend of Objective-C++, Python, and Haskell all mixed together. Swift offers developers the ability to take advantage of a wide set of Objective-C APIs, types, and control flow, while still providing objects, inheritance, and protocols that are familiar from Objective-C and C++. For example:
Interface declaration of a Queue type in Objective-C:
@interface Queue : NSObject @end
Object declaration of Queue type in Swift:
Class Queue : NSObject { }
From a Python perspective, Swift offers a unique level of type inference to allow types to be inferred at runtime. Developers can use type inference or strongly declare variables statically for optimized performance. For example, an excellent use case for Swift’s type inference is setting properties from data parsed from a web service. For example:
let firstName = jsonObject["firstname"]
Or if you wanted to strongly declare a mutable type as a string you could declare a property that looks something like this:
var firstName: String = "Matt Eaton"
From a functional perspective, in the context of a language like Haskell, Swift offers many functional methods like map and filter. For example:
var numbers = [1, 2, 3] let mappedValues = numbers.map ({(value: Int) Int in return value * 4 }) // produces mappedValues: [4, 8, 12]
One of the most unique features of Swift and probably the biggest selling points to me right now is Swift optionals. I personally used to hate Swift optionals, but after I started dealing with a lot of data processing and manipulation the applications I was building, I really began to love them. A Swift optional is a way to carry around nil safely in the language without getting a compile time error. In Swift, you cannot assign a mutable or constant property a nil value because Swift’s compiler will throw an error back at you. Instead, what you can do is create an optional to set a nil value safely, or carry around a value around in that property if you want. The tricky part about using optionals for developers coming into Swift is understanding how to unwrap optional properties safely. For example, here is how you create an optional:
var firstName: String? // firstName is carrying nil safely firstName = "Matt Eaton" // firstName is now carrying a string value of Matt Eaton // Now to access the value of Matt Eaton the optional must be unwrapped safely if let name = firstName { print(name) } // The guard control flow can optionally unwrap and set a property or defer logic elsewhere guard let name = firstName else { return } print(name)
Swift’s approach to concurrency shares a similar pattern with Objective-C because Swift is still built on top of the Objective-C runtime. Swift uses grand central dispatch for processing concurrency or asynchronous operations. For example, let’s say that I want to execute a couple of long running tasks on a background thread and then notify the main thread once these operations have finished to update the user interface. The approach in both languages is to dispatch an operation into a background thread’s queue, often referred to as the global queue. Once the global queue has time to execute these operations, they are executed in the order in which they were placed into the queue. This action frees up the main thread’s queue to process user driven events concurrently while the background queue is processing and does not have to be distracted by operational events that may need to run in the background. For example:
DispatchQueue.global(qos: .userInitiated).async { // Execute long running operation here in a closure DispatchQueue.main.async { // Execute a main thread operation on the completion } }
Swift’s Major Application in Today’s Development Environment
Swift’s major application in today’s development environment has really evolved from where Objective-C allowed the Apple Developer community to be. For example, it used to be that if you were writing Objective-C that meant that you were writing iOS or macOS applications because that was the only marketable use case for the language. Well, from the very day Swift was released, the Swift language was also available on Linux as well. This means that anyone wishing to now write Swift for Linux could possible write Swift on the web as well. As many companies started to realize the opportunity here, there has most certainly been a strong movement into developing server side Swift so that your iOS app and web application can now all run with certain shared components of a code base. IBM’s Kitura and Qutheory’s Vapor are among those companies making a large impact with server side Swift.
Along with Swift on Linux, 2014 was also the year that Apple announced two new platforms, tvOS and watchOS to run their two new product lines for the Apple TV and the Apple Watch. So the major applications for Swift have certainly spread out in the last couple years and they're much more of a surface area for Swift development than there ever was for Objective-C.
Swift’s Major Strength and Weaknesses
First let’s talk about the weaknesses of Swift. Depending upon who you talk to, Swift has many weaknesses. However, I feel that instead of muddying the waters here and giving my opinion, I will try and focus on the larger elephants in the room and that is most certainly language and ABI stability. What is an ABI? An ABI is an application binary interface. It is the entry point for all other languages communicating with your Swift compiled code. As of right now the ABI stability is pretty fragile and that does not make for adoption across many other languages and operating systems. A fragile ABI is a good way for a language to die on the vine and not gain widespread adoption like languages such C++ and Java have.
The next weakness is language stability. As I mentioned earlier, Swift has been going through some massive changes in the last two and a half years and to tell you the truth, developers have had enough of it. I know I have. My team at work just finish a Swift project that is probably close to a quarter of a million lines of Swift. Thankfully this code base is entirely in Swift 3 and we did not start it in anything below Swift 3. Swift 3 is when things finally started slowing down a bit as far as massive breaking changes, but from Swift 1 to Swift 3, developing any kind of stable project with Swift was a rocky road.
The major strengths of the Swift language I feel are safety, static or inferred types, and interoperability with Objective-C.
Now let’s talk about the strengths of Swift. Swift has many strengths in my opinion, but like I did with the weaknesses I will only focus on the large well known strengths as to not muddy the waters. The major strengths of the Swift language I feel are safety, static or inferred types, and interoperability with Objective-C. First let’s talk about safety, as I mentioned earlier, Swift has optionals, and optionals make it really hard to use nil incorrectly and cause a crash in your application due to the usage of nil. This may not seem so impressive from a developer who is coming into Swift, but from a developer who spent years in Objective-C, the options feature in Swift is an excellent feature to have.
The next strength is static or inferred typing. This is interesting, is it not? Swift gives the developer the option of declaring the type for speed and safety or letting the compiler infer the type for you. In a development era dominated by interpreted languages I think this was a great move to drive adoption into a compiled language that gave developers the ability to use both options. Type inference is also excellent because it removes the need to write the boilerplate code to do this by hand. This was often the case in Objective-C, that there were all sorts of ugly code that we had to write as Objective-C developers to make sure that data that was coming in from the web and was processed properly.
Lastly as a strength of Swift, let’s talk about Objective-C interoperability. So what does this mean? This means that as a developer I can take my large Objective-C, C++, and C codebase that I spent years writing and bridging in new features from Swift as I convert the C code to Swift. This gives developers the flexibility to work in both code bases at the same time. So how does this work? Well, often a good place to start is by writing a user interface in Swift and then creating a C bridging header to expose the C based code to the Swift through a module importing. That way if Swift ever needs to interact with any of the C based code, it can just make function calls down to it and interact with it just as Objective-C used to.
Summary of the Swift Language
In summary, I am very excited about how the Swift language has grown over the last couple of years and I am very excited to see where it goes in the years to come. If there was one thing that makes me very excited about working with Swift it is that Swift can be there to write the entire application side of a project. Then if there is any heavy computation or extensive processing to perform, then Swift will give you the ability to still interact with Objective-C and Objective-C++ if needed. This has me very excited and I cannot wait to see where Swift goes from here. Please let me know what you think by leaving a comment, question, or correction if you see one. Thank you very much for reading!