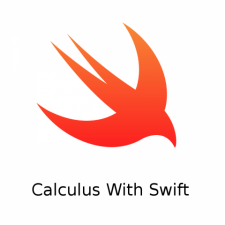
The other day on Twitter I seen mention of the Taylor Series and it made me think back to calculus and how much I enjoyed the Taylor and Maclaurin series because learning about them was my first exposure into series and sequence approximations. Thinking about how the Taylor Series expansion works and how epanding it is essentially the same polynomial expanded by the order of the derivative immediately made me think that I could write a program that represented this calculation out to the order of X pretty easily!
To write my program I decided to open up an Xcode Playground to build my Taylor Series. Xcode Playgrounds are really really nice for testing out programs and ideas because Playgrounds allow you to create a small, or if needed, a fairly graphical program without hardly any hassle in getting your program setup. So to get my Playground started all I needed was a Taylor Series to calculate. Looking around, I decided to go onto Khan Academy and build my Playground based off of the series e^x when x = 3 that is explained in this video with a graphical representation of the series from Wolfram Alpha. The idea for my series expansion was that I would take the first value in the polynomial, e^3, add the decimal value to the series array, and then use a loop to generate the rest of the decimal values out to the order of five.
After I generated all of my decimal values for my series out I needed something to validate them against to show me from a visual perspective that I was on the right track. Below you will see a screen shot from a Jupyter Notebook that I created to plot out the decimal values generated in my Playground and verify that the graph in my Jupyer Notebook was the same as the one that was being displayed in Wolfram Alpha. Success, the two graphs are pretty darn close!
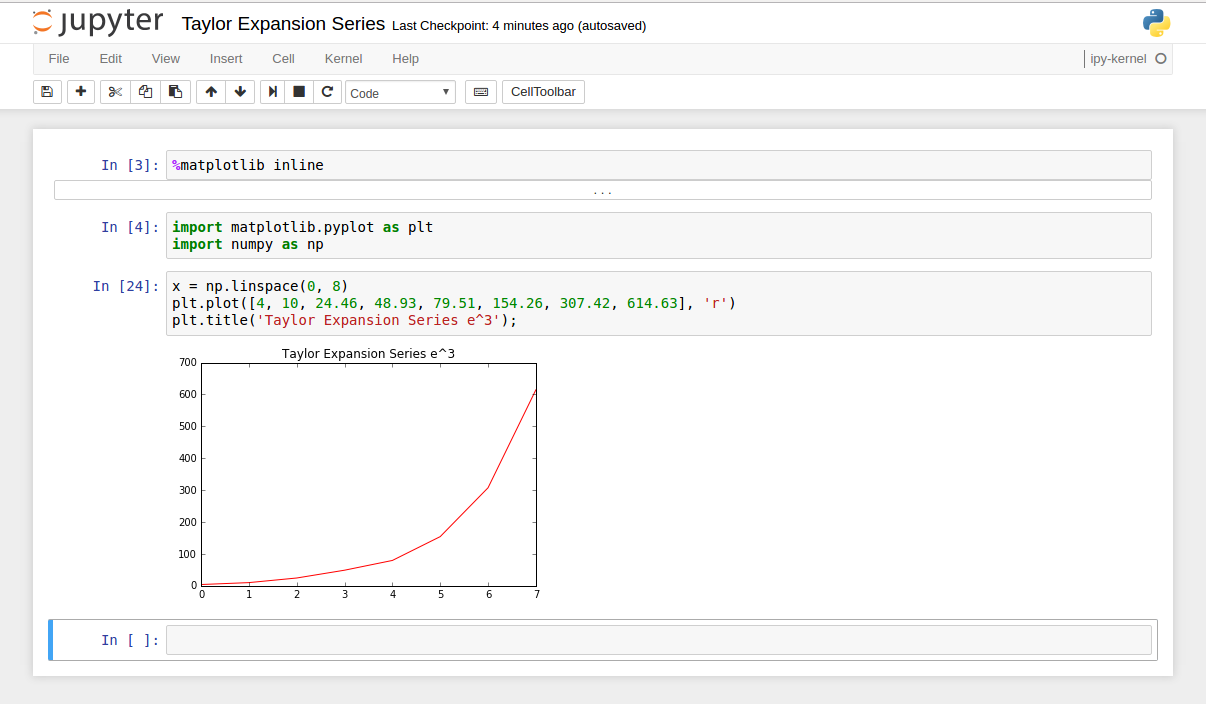
Below is the code from the Playground I created for the Taylor Series e^x where x = 3 in Swift.
/** * Taylor Series Approximations about x=3 up to order 10 * * Based upon inspiration from: * https://www.khanacademy.org/math/integral-calculus/sequences-series-approx-calc/taylor-series/v/visualizing-taylor-series-for-e-x * https://youtu.be/AFMXixBVP-0?t=174 * */ import Foundation /** * Factorial generator */ func factorial(f: Int) -> Int { // Make sure we are not calculating factorials for 0 if f == 0 { return 0 } var f = f for i in stride(from: f, to: 1, by:-1) { f *= i } return f } /** * Exponent generator */ func exponent(v: Double, power:Int) -> Double { // Make sure there is no upper bound issues if power == 0 { return 0 } var v = v for _ in 1..<power { v *= Double(power) } return v } /** * Taylor Series expansion at x = 3 * Wolfram Alpha Link for Taylor Series of e^x when x = 3 * https://www.wolframalpha.com/input/?i=taylor+series+e%5Ex+x%3D3 */ func taylorSeriesApprox(x: Double) -> Void { // Set numerical value for e let e: Double = 2.71828 var approx_polynomials: [Double] = [] // Add in e^3, this is the first term in the series let zero_value = exponent(v: e, power:Int(x)) approx_polynomials.append(zero_value) // Generate the decimal approximation up to order five for index in stride(from: 1, to: 6, by:+1) { // Step 1, get the power // e^3 let power:Double = exponent(v: e, power:Int(x)) // Step 2, get the factorial // index! let fact:Double = Double(factorial(f: Int(index))) // Step 3, get the parenthesis value // (x - 3) // Mostly useless, but part of the equation var paran:Double = Double((x - x)) // Step 4, apply exponent to parenthesis value // (x - 3)^index // Equals zero almost always paran = exponent(v: paran, power:Int(index)) // Compute decimal approx at order (index) var orderValue:Double? if paran != 0 { orderValue = ((power / fact) * paran) } else { orderValue = (power / fact) } for val in approx_polynomials { orderValue = orderValue! + val } approx_polynomials.append(orderValue!) } var i:Int = 0 for v in approx_polynomials { print("Decimal Approx: \(round(100*v)/100) at order \(i)") i = i + 1 } } // Approximate the decimal values greater that 3 when x equals 3 var x: Double = 3.0 taylorSeriesApprox(x: x)
You can find a copy of this Playground in my Github account here. Please take a look and submit a pull request if I missed anything or made a mis-calculation anywhere in my computation. Also, if you have a question, comment, concern about this Playground, please submit it to the site and let me know. I would love to hear from you! Thanks.