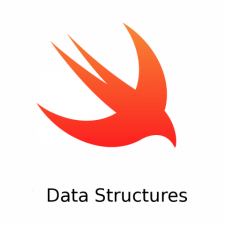
Today I was analyzing a potential bottleneck in some computation I was doing with Swift and thought I would try a basic linked list data structure to store data instead of an array. It soon dawned on me that I have never created a linked list in Swift before and thought this might be a good opportunity for a Swift Playground to test out my idea. Sure enough, using Playgrounds was a great way to test out my code and make sure it was running the way I wanted it to before integrating it into my project.
Below is an example of my basic linked list data structure. In this example I just create a basic class instead of the stradition struct and then build my list using an array of integers. Usually the data loading into a data structure like this is coming from a data table or a network and then formatted specifically in a linked list to serve some purpose, so the array of values is not your typical scenario. Then I created a function print the list iterating all the way from the head to the tail. Let me know if you have any feedback, thoughts, or improvements for my example. The source code can be found on my Github here. Thanks!
// Linked List Playground //: Playground - noun: a place where people can play // // @Author, Matt Eaton // import Foundation // Node Data Structure // Could be a struct but I used a class class Node: NSObject { var data: Int? var next: Node? init(d: Int) { super.init() self.data = d self.next = nil } } // // Function to get the head and build a list from an array of values // // @param dataList // Array of integer values for the list // @param head // Head of the list // @return the build list // func buildList(dataList: [Int], head: Node) -> Node { var current = head for index in stride(from:0, to:dataList.count, by:+1) { let newNode = Node(d: dataList[index]) current.next = newNode current = newNode } return head } // // Function to print the head starting with the first value // // @param head // Head of the list // @param len // Integer value length of the list // @return the build list // func printList(head: Node, len: Int) { var h = head for i in stride(from:0, to: len, by: +1) { print("Data: \(h.data!) at index: \(i)") if let newHead = h.next { h = newHead } } } // Array of integers var data = [3, 4, 1, 5, 6, 10] // The first element in the list var head = Node(d: 7) // Build the list with all of the other values head = buildList(dataList:data, head: head) // Print the list printList(head:head, len: (data.count + 1))