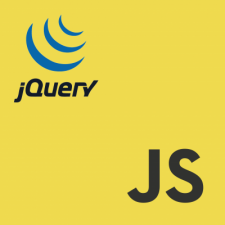
To illustrate the ease of using vanilla JavaScript over jQuery I decided to create a list of five examples that would demonstrate parallel behavior between JavaScript and jQuery. The examples in this list are intended to be real world examples, but if you have any other examples I would love to hear about them too!
Another great resource to compare JavaScript and jQuery code samples is You Might Not Need jQuery.
Applying CSS to an element.
<h1 id="exampleOne">Styling</h1> ... // jQuery $('#exampleOne').css({ 'background-color': 'red', 'padding': '10px 0' }); // Vanilla JavaScript var h1 = document.getElementById('exampleOne'); h1.style.backgroundColor = 'red'; h1.style.padding = '10px 0';
Getting data attributes from an element.
<h1 id="exampleTwo" data-text="Custom Data">Get Data Attribute</h1> ... // jQuery var dataText = $('#exampleTwo').attr('data-text'); // Vanilla JavaScript var dataText = document.getElementById('exampleTwo').getAttribute('data-text');
Getting classes from an element.
<h1 id="exampleThree" class="classOne classTwo">Get Class Attribute</h1> ... // jQuery var classValue = $('#exampleThree').attr('class'); // Vanilla JavaScript var classValue = document.getElementById('exampleThree').className; console.log(classValue); // Prints out: classOne classTwo
Creating a custom element.
<div id="exampleFour"></div> ... // jQuery $('<div/>', { id: 'vanilla', title: 'Learn Vanilla JavaScript', text: 'Agnostic Dev!' }).appendTo('#exampleFour'); // Vanilla JavaScript var sample_div = document.getElementById('exampleFour'); var vanilla_div = document.createElement('div'); vanilla_div.setAttribute('id', 'vanilla'); vanilla_div.setAttribute('title', 'Learn Vanilla JavaScript'); vanilla_div.textContent = 'Agnostic Dev!'; sample_div.appendChild(vanilla_div);
Getting a parent element.
<div data-text="parent"> <p>Child Node</p> </div> ... // jQuery var parent = $('p').parent().attr('data-text'); // Vanilla JavaScript var parent = document.getElementsByTagName('p')[0].parentNode; console.log(parent.getAttribute('data-text')); // Prints out: parent