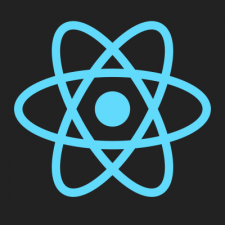
React JS is a semi-new open source JavaScript rendering library that came out of a collaboration between the Instagram and Facebook engineering teams. React was created as better a way to approach complex user interfaces where the traditional MVC approach was just not sustainable anymore. Implementing React components and Flux style data patterns attempts to solves these complex issue by providing modularity between user interface components and a one-way flow of data on the client side instead of always interacting with the server, as is done in an MVC approach. Flux is a relatively new way to think about how to implement data into your application that works closely with React. The benefits that came from using Flux patterns and React components greatly reduced the complexity of client side applications at both Facebook and Instagram and now React components are used throughout both companies entire front end stack.
There are a couple of great talks on this subject that came out of React JS Conf and F8 earlier this year. One being a keynote from Tom Occhino on how React was started and how it is used at Facebook today. Another from Jing Chen about Flux, what it is and how it is used in conjunction with React. Below I included the entire talk from F8 that included talks from Tom Occhino, Jing Chen, and Pete Hunt that talk about React and Flux.
Now that I laid the groundwork for what React is and where it came from, let me tell you a little bit about how I have been using React. I have been using React a lot lately to try and reduce the complexity between my client side JavaScript, my markup, and the server side side code. As an example, I built an application that uses Node.js as the server, EJS as the template library, and I was using jQuery to handle client side interaction. So the workflow went, I would query my data in Node.js, send it down to the view where I would use EJS to disperse my data throughout my HTML elements, and where I needed client side interaction, I would create a basic jQuery plugin to handle this interaction. Sounds easy enough right? Well, this is fine if you have a very basic web application. As I started to develop more and more complex features the site code quickly became tangled and messy. I actually finished almost all of the extremely complex development on the application before I took a hard look at how sustainable the code might be in the future to maintain and add onto if needed. I knew I was in a bad spot, so I started looking at React to develop small portions of the site to try and reduce complexity piece by piece. This worked really well. I started creating small components and soon I wanted to create whole sections using React. It was not long until I had taken all of the logic and data implemented by Node.js and EJS and moved it to React. All I pretty much needed Node.js do is process authentication and serve pages. The beauty from using React is that I can load my data, write my templates, and process client interaction all in the same workflow. I do not need three separate pieces of my application to perform these actions.
Below is an example of using React to pass data from a parent element to a child element. This example is fairly trivial but what it does is it demonstrates a couple of things. The first thing it demonstrates is how modular components can be setup to interact together. The second thing it demonstrates is how the markup, data, and client interaction are all processed within the context of React. This was one of the major benefits I seen in React based upon the application I most recently overhauled. Using React encompassed all the elements needed to create a rich application. I did not need to go to each technology and built out these interactions.
Lets talk a little bit about the text/jsx type on my script tag and the JSXTransformer. JSX , taken straight from the React docs, is a JavaScript syntax extension that looks similar to XML. You can use a simple JSX syntactic transform with React. What that means is that you can write your markup right in your React components and JSX will transform them into raw JavaScript when the page is loaded. I personally prefer to use a build step to do this, so that I do not have to transform them when the page loads, but so that the script that is delivered to the browser already has a tree a transformed JavaScript.
<!DOCTYPE html> <html> <head> <script src="https://fb.me/react-0.13.1.js"></script> <script src="https://fb.me/JSXTransformer-0.13.1.js"></script> </head> <body> <div id="test"></div> <script type="text/jsx"> var ParentNode = React.createClass({ render: function(){ var returnContent, self = this, textData = [ {id: 1, data:'child one', title:'Parent One'}, {id: 2, data:'child two', title:'Parent Two'}, {id: 3, data:'child three', title:'Parent Three'} ]; var returnContent = textData.map(function(object){ return ( <div className="row"> <h3>{object.title}</h3> <ChildNode key={object.id} childData={object.data} /> </div> ); }); return (<div>{returnContent}</div>); } }); var ChildNode = React.createClass({ getInitialState: function() { return { textData: this.props.childData}; }, render: function(){ return ( <div className="childNode"> <div>{this.state.textData}</div> <button onClick={this._handleClick}>Update</button> <hr /> </div> ); }, _handleClick: function(){ var newText = 'Updated Text!'; this.setState({ textData: newText, }, function(){ //notify the parent if needed }); } }); React.render( <ParentNode />, document.getElementById('test') ); </script> </body> </html>
React has become a very popular library over the last six to eight months. If you find what you have read here intriguing or you want to know more about React I encourage you to checkout their GitHub page and GitHub docs and consider getting involved in contributing. Likewise if there is something you have questions on or want to see more examples of React, let me know and I can update this post with some broader examples of possibly using React to load data via Ajax or loading data in React via Parse.