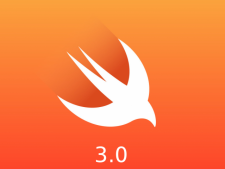
At the time of writing this Swift is the number one language project on Github. Apple is very proud that Swift is open source and I would have to agree that this is an excellent step forward for the language and for Apple. Another great step forward from a language and platform perspective is the ability to run Swift on macOS, iOS, watchOS, tvOS, and Ubuntu Linux. This is very interesting because it displays the the commitment on Apple's part to drive the language forward and gain support from the C community on Linux as well. Over the last 2 years Swift has already changed a lot and version 3.0 is no different. Since early December of last year, in preparing for Swift 3.0, Apple has said that their main focus for Swift was to improve the stability and maturity of the language with this next release. This is an excellent goal, but in my opinion, this is a little out of context still because if you have been following the Swift Evolution mailing list or the Github project you will know that there are a lot of big syntax changes to Swift 3.0 and this maturity and stability of the language is not planned for the development jump from Swift 2.3 to 3.0 but from Swift 3.0 to 3.x or 4.0 instead. Maturity and stability are coming, Swift 3.0 is just laying the groundwork for this to start happening.
Swift 3.0 has a lot of syntax and type changes to the way you write Swift. Some of these changes focus around moving away from the traditional NS prefix found in the typical Foundation class primitives and also shortening up the syntax of Swift 2.2 API calls to be a lot easier to chain, read, and understand while you are developing. Below I have outlined some of the major code changes demoed at WWDC and provided in the form of proposals from the Swift Evolution Github project as a way to demonstrate these new changes.
New Type Declarations and Shortening of APIs
New type declarations and the shortening of APIs between Objective-C and Swift can be found throughout multiple proposals (SE-0005, SE-0023, SE-0006) and also highlighted in the state of the platform talk at WWDC this year. Most of the information found in these proposals centralizes around making sure that Objective-C to Swift API stay consistent but also become less verbose and easier to type and remember on the fly. While shortening verbose API calls is pretty significant, another significant change that was made was new type names to some of the traditional types that used the original Foundation prefix of NS. Below are two examples, one of the new NSDate type and one of the new syntax for Grand Central Dispatch that was demoed at the state of the platform, looks a bit like JavaScript, does it not?
// New Swift NSDate var myDate = Date() // Here was another demo from the state of the platform using the new Grand Central Dispatch syntax let queue = DispatchQueue(label: "com.vendor.queue") queue.async { // perform action }
Remove the ++ and -- operators - SE-0004
Removing the increment and decrement operators from Swift is a very interesting change. These operators carry over C style syntax from most languages like Java, Objective-C, C++, or C#, and most developers coming from these languages would recognize these operators right away. However in this proposal some of the disadvantages to keeping these operators in Swift include the burden this puts of new developers to learn these operators, the shorthand expressiveness of the operator is only 2 characters off from the original syntax (i = 1 + 4), and probably the the most compelling argument to me is that this operator in Swift deviates from C because it returns void instead of zero. To help illustrate this point in C and in Swift I included two snippets below. The first from C that adds the value of f to d and then reassigns d and assigns f to d. Using gcc, this example compiles and outputs 0 on the second assignment even though f is not initialized with a value.
#include <stdio.h> int main(void) { int f; int d = 4; d += f; printf("D %d\n", d); d = 4; d = f; printf("D %d\n", d); return 0; } // The output of this is: // D 4 // D 0
Using this same example in Swift 3.0 Preview 1 on Ubuntu 15.10, Swift will not even compile because of the error that says that F is used without initialization. To me, this is a good example of the deviation between C and Swift.
import Glibc import Foundation var f:Int; var d:Int = 4; d+=f; print("D \(d)"); d = 4; d = f; print("D \(d)");
Remove C-style for-loops with conditions and incrementers - SE-0007 - Community Submitted
A very interesting proposal from Erica Sadun is the proposal to remove C-style for-loops from Swift 3.0. I will admit, at first look, I was not sold on this proposal. After all, C-style for-loops have been in use in all major languages that I have ever wrote except Python and possibly Visual Basic way back when. I think that this skeptical reaction is the first thought for most other developers looking at this proposal too, especially those coming from Objective-C, C, and C++.
After completely reading this proposal there are sublte points being made about for-loops and how they are not really contributing to making Swift a better language that are quite compelling. There is an interesting point that reads if for-loops never existed at all, then for-loops probably would not be considered to be added to the language at all. Think about that for a minute. That is really interesting point because if for-loops never existed and there is already constructs that mimic for-loops already in Swift, who would think that adding them as a new feature would be a benefit the language? The last interesting point I took away from this proposal is that the whole reason for-loops are in the language is because they are a left-over from C and everyone feels comfortable with them.
Here is an example of a C for-loop:
// Compiled with gcc int array[5] = {10,20,30,40,50}; int i; for (i = 0; i < 5; i = i + 1 ){ printf("array[i] %d\n", array[i]); }
Here is an example of a new for-loop in Swift 3.0 without the C-style syntax on Ubuntu 15.10
// Compiled and ran on Linux var array = [10,20,30,40,50] for var i in 0..<array.count { print("array[i] \(array[i])") }
Removing var and let from Function Parameters - SE-0003 and SE-0053 SE-0053 - Community Submitted
I decided to describe both proposal SE-0003 and SE-0053 in the same section as they both deal with the same topic. An initial discussion was brought up on whether or not adding the var keyword was confusing because writing a function definition with and without the var keyword were both valid as well as mutable whether the var keyword was there or not. So it was decided that the var keyword would be dropped from function definitions and from there the let keyword followed suite as to stay consistent with dropping var. Below is and example of both.
// Function with var in the definition func functionWithVar(var x: Int) { // Perform Functionality } // Function without var in the definition func functionWithOutVar(x: Int) { // Perform Functionality } // Function with let in the definition func functionWithLet(let x: Int) { // Perform Functionality } // Function without let in the definition func functionWithOutLet(x: Int) { // Perform Functionality }
Standardize function type argument syntax to require parentheses - SE-0066
I think that proposal SE-0066 to standardize function argument syntax is a great proposal and removes allowed inconsistencies that I was unaware even existed. So while this is not a tremendous benefit to upgrading features of Swift it certainly makes the language a lot more consistent. This proposal points out that if writing function type syntax with no parentheses is simple a feature that is syntax sugar in the language and does not provide anything the that enhances the consistency or expressiveness of the language. In my opinion this is great, especially for new developers coming in and learning the language it provides consistency when they look at code examples or projects out in the wild that may be using both forms of this syntax interchangeably. Below is a simple example illustrating both versions of this syntax.
// Function without using parentheses func myFunction x: Int { // Perform Functionality } // Function with using parentheses func myFunction(x: Int) { // Perform Functionality }
These are certainly not all of the new changes that are coming in Swift 3.0, but they are the ones that I considered worth mentioning. If you would like to check out all of the new features coming to Swift 3.0, head on over to the Swift evolution Github page and take a look at the implemented proposals for Swift 3.0. Also, one last thing worth mentioning, at WWDC it was announced that Xcode 8 would still carry support for Swift 2.3 and Swift 3.0 still and it would not be an immediate drop in support, so this is good if there are syntax changes you need to make to your project to support Swift 3.0. Please let me know if you have any questions, comments, or concerns, I would love to hear from you!