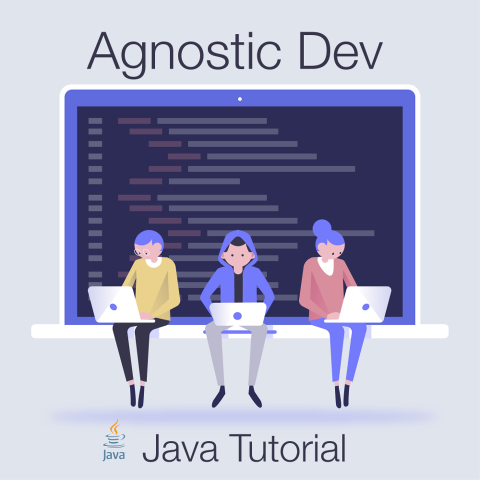
Java is a very concrete and powerful language. In the last 20 years Java has become a staple in enterprise, mobile, and server side computing. However, if you're coming over to Java from languages like Swift, Python, or Haskell you might say be wondering about different functional aspects of Java. One question I had is, "How come Java does not support n-value heterogeneous return type tuples?" The answer is that Java's methods are intended to have one return type per method call. If multiple return types are desired, then this would be a call break up these pieces of data into different methods calls or wrap these types in a custom object. Coming to Java from a more functional language can be a mind shift in this way, but in Java's case it's a shift that makes sense when thinking about how the language works. So in this tutorial I wanted to provide two examples of returning custom objects and lists from a method call, instead of a tuple. Let's jump in!
Returning Custom Objects 🚀
First let's look at a technique to return heterogeneous types and that is of course wrapped in an object. Returning a custom Java object from a method is a staple of Java programming and can be used to return multiple types or the same set of types. One thing to note is that these types in Java all conform to the same context. Let's take a look at for example the ErrorWrapper custom class below. This class takes in a custom message and error code. Two different types, but all from the same context. This allows the custom object to be created and returned from a method call to be used throughout your program. Here is an example below:
public class ErrorWrapper { private String message = ""; private Integer errorCode = 0; public ErrorWrapper(String m, Integer c) { message = m; errorCode = c; } public String getMessage() { // Do some logic here based upon error code and message return message; } public Integer getErrorCode() { return errorCode; } } public ErrorWrapper reasonAboutErrorException(Exception e) { ErrorWrapper errorWrapper = new ErrorWrapper(e.getMessage(), 100); // Perform some logic on errorWrapper return errorWrapper; }
Returning List Types 📝
The heterogeneous object is probably a bit more work, but returning a set of homogeneous types can be a lot more straight forward. In a case where multiple strings or integers are needed, a simple Java list is all that is needed to be assembled. A Java List is nothing more than a collection or an array of same types assembled in a method call and returned accordingly. For example see the following code below:
public List <String> reasonAboutException(Exception e) { List <String> returnStrings = new ArrayList <String>(); returnStrings.add(e.getMessage()); returnStrings.add(e.getCause().toString()); return returnStrings; } List <String> inferredExceptionList = reasonAboutException(e); String string1 = inferredExceptionList.get(0); String string2 = inferredExceptionList.get(1);
In Summary ⌛️
I hope this short tutorial was helpful in learning more about returning objects and lists in Java instead of tuples. Java is a great language and is a cornerstone of object orientated programming. One might argue that there is no need for using tuples in Java, and I would to agree. Only time will tell if these more functional aspects are integrated into the language at over time. I hope you enjoyed this and if you have any questions, comments, or concerns, please leave a comment below and I will try to respond as soon as possible. Thank you!