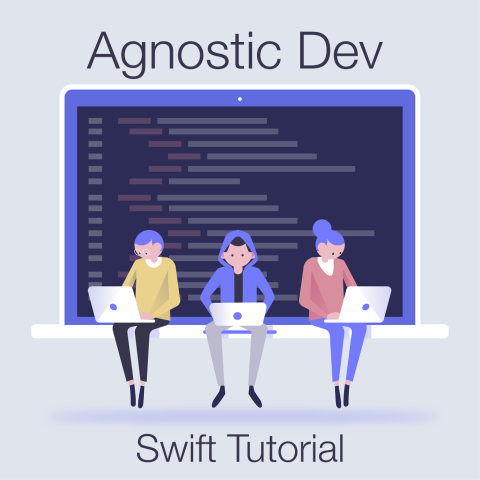
If you have ever wondered how to find a substring inside another string in Swift then hopefully this tutorial will help walk you through how to do that. As an example, in Swift 3 or above, if you have ever wondered how to determine if the substring "Agnosticdev," is part of the parent string "Hello Agnosticdev, I love Tutorials," then this tutorial will show you how to indicate, with a boolean value, the existence of the substring. This tutorial takes advantage of the Swift's String Standard Library API reference, for working with and manipulating Strings, and it would be good to brush up on these API's before reading further.
NOTE: This tutorial assumes that you are at least using Swift 3 or greater. Swift 2.3 or below has not been tested, only Swift 3.0 and Swift 4.0. This tutorial is recommended for all Swift users, even beginners, and takes advantage of Swift Playgrounds to demonstrate the code used from this post. If you would like a copy of the playground, please download it here from my Github page.
Code Example:
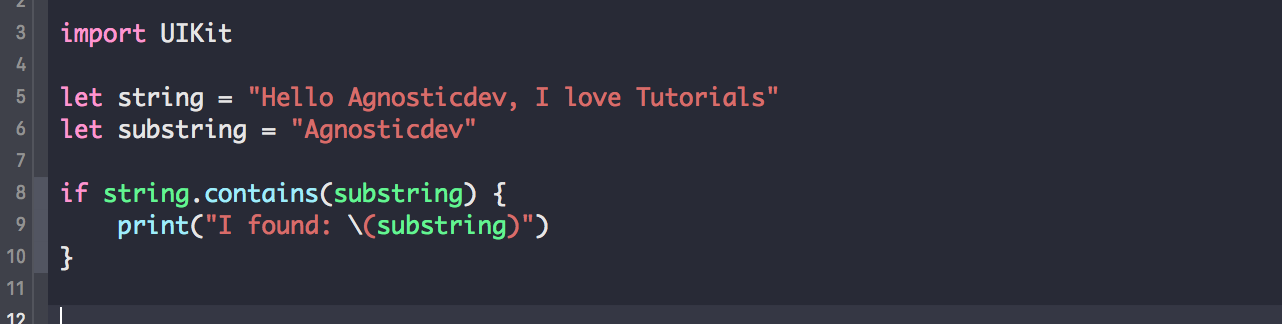
Utilizing the contains instance method from the String class, out of Foundation, will return true if a range of a string is contained within another string. As an example, if a parent string was iterated through like a collection of characters and then a range of a substring was checked for at each character in the parent string, this could be a conceptual example of how finding a substring inside a parent string works.
let string = "Hello Agnosticdev, I love Tutorials" let substring = "Agnosticdev" if string.contains(substring) { print("I found: \(substring)") }
Alternative Approaches:
An alternative approach from using the contains method would be to search for the first character of the substring in the parent string and then attempt to cut the substring out of the parent string based upon a dynamic range that is created based upon the matched character index.
Download the Swift Playground used in this tutorial, here on Github.
// Alternative Options let string = "Hello Agnosticdev, I love Tutorials" let substring = "Agnosticdev" var index = 0 // Loop through parent string looing for the first character of the substring for char in string.characters { if substring.characters.first == char { // Create a start and end index to ultimately creata range // // Hello Agnosticdev, I love Tutorials // 6 -> 17 - rage of substring from 7 to 18 // let startOfFoundCharacter = string.index(string.startIndex, offsetBy: index) let lengthOfFoundCharacter = string.index(string.startIndex, offsetBy: (substring.characters.count + index)) let range = startOfFoundCharacter..<lengthOfFoundCharacter // Grab the substring from the parent string and compare it against substring // Essentially, looking for the needle in a haystack if string.substring(with: range) == substring { print("Found: \(substring)") break } } index += 1 }
Now you know more about how to find substrings in parent strings in Swift! As always, if you have a comment or questions please feel free to reach out to me or leave a comment.
Thank you very much for reading!