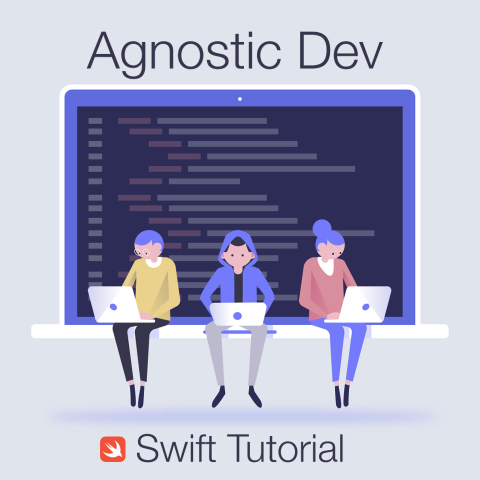
Have you ever found yourself trying to remember the syntax on how to grab the first few characters from the start or end a string in Swift 4. Well, I certainly have from time to time and that is why I wanted to write this tutorial. To show you the reader how to grab the first few characters from the start or the end of a Swift string using only two Swift Standard library methods, and from there show one more slightly advanced technique. So by the end of this tutorial you should be able to get the first few characters from the start or the end of a string in either Swift 3 or 4.
NOTE: This tutorial was created using a Xcode 9.2 Playground and assumes you have at least Swift 4.0 installed in your Xcode toolchain or that you are using a version of Xcode that supports Swift 4, such as Xcode 9. To get started with this tutorial you can know a lot about Swift or a little about Swift, these examples are aimed at everyone. 👍
Getting Started 👨💻
Let's get started by providing our sample string, "Hello, Agnostic Dev." Let's say that our goal is to grab the first two or the last two characters from this string. Well, to achieve this, the Swift team has created the prefix(_:) and suffix(_:) methods. These APIs work exactly like they sound in that the prefix method grabs the nth characters off the start of the string up to the passed in integer and the suffix method does the same off of the end. If the passed in integer is greater than the collection, then the whole collection is returned. The beautiful part about this is that strings are not the only collection that these instance methods work on, arrays work as well!
var str = "Hello, Agnostic Dev" var charArr: [Character] = ["A", "g", "n", "o", "s", "t", "i", "c"] // Grab the first two characters if str.prefix(2) == "He" { print("I found \(str.prefix(2))") // I found He } // Grab the last three characters if str.suffix(3) == "Dev" { print("I found \(str.suffix(3))") // I found Dev } // First three from charArr: ["A", "g", "n"] print("First three from charArr: \(charArr.prefix(3))") // Last three from charArr: ["t", "i", "c"] print("Last three from charArr: \(charArr.suffix(3))")
Alternative Approaches 🚀
Alternatively, a slightly more advanced approach would be to create new instance methods as an extension of a Swift String. This would allow you to define your approach for grabbing characters on a string. For example, in the extension created below character(at: position) is defined on the string to pluck certain characters from your string at a position. If the position passed in is too large for the collection this will result in a nil value being returned and thus the character cannot be unwrapped in the if-let statement.
// Build your own String Extension for grabbing a character at a specific position extension String { func index(at position: Int, from start: Index? = nil) -> Index? { let startingIndex = start ?? startIndex return index(startingIndex, offsetBy: position, limitedBy: endIndex) } func character(at position: Int) -> Character? { guard position >= 0, let indexPosition = index(at: position) else { return nil } return self[indexPosition] } } if let character = str.character(at: 3) { print("I found \(character)") // I found l } if let character = str.character(at: 31) { print("I found \(character)") // Will not return due to a position too large }
In Summary ⌛️
Now you know more about how to grab the first or last few characters from a Swift string using a Standard Library API or a more advanced approach! As always, if you have a comment or questions please feel free to reach out to me or leave a comment. You can download the playground used in this tutorial on my GitHub here.
Thank you very much for reading!