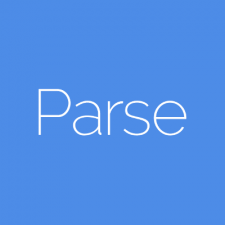
In the last couple of years I have been involved with a lot projects all with the same type of scenario, there is a server housing a content management system or a database, a remote mobile application that reads data from the server, and usually a web application for anonymous and authenticated web traffic. Projects like this are extremely interesting, but can get very complicated in a hurry as the project evolves and features are added on either the web side or the mobile application side. Suddenly, you are in a situation where actions on the web application start effecting the user experience on the mobile application, and vice versa. So what do you do? Keep developing addition layers to your network stack and your data feeds? Keep increasing the codebase and the complexity of the project for those "one-off" features? Well, yes, what other choice did we have? The project needed to keep evolving and features needed to be developed. This led me and my co-workers down a road that we knew one day could lead to issues if we had to bring new developers up to speed on the projects. It was not until one of my co-workers suggested that we look into Parse that I could see a resolution to this issue anytime soon.
What is Parse and how does it work?
Parse is a cloud based data store that hosts all of the your project data in a NoSQL backend and then exposes that data to a variety of different platforms using one of their extensive SDKs. So if you have a mobile and web application that you need to setup, you simply create a project in Parse, configure your class objects, and then download the SDK to start development. This process in Parse replaces the need to setup and configure servers, develop network stacks in your mobile application, and develop your data feeds from either your content management system or your database. Parse will handle all of the data synchronization for you so that all data that is delivered to any platform is the most up to date data available.
As an example, the following code shows how I would query the same set of data between a web and an iOS project.
PHP
$query = new ParseQuery("GameScore"); $query->equalTo("playerName", "Matt Eaton"); $results = $query->find(); // Do something with the returned ParseObject values for ($i = 0; $i < count($results); $i++) { $object = $results[$i]; echo $object->getObjectId() . ' - ' . $object->get('playerName'); }
Objective-C
PFQuery *query = [PFQuery queryWithClassName:@"GameScore"]; [query whereKey:@"playerName" equalTo:@"Matt Eaton"]; [query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) { if (!error) { // The find succeeded. NSLog(@"Successfully retrieved %d scores.", objects.count); // Do something with the found objects for (PFObject *object in objects) { NSLog(@"%@", object.objectId); } } else { // Log details of the failure NSLog(@"Error: %@ %@", error, [error userInfo]); } }];
The Parse SDKs are pretty extensive. Here is a list of the languages and platforms that are available:
- Objective-C, OS X, iOS.
- Java, Android.
- JavaScript, client side JS and server side JS via Cloud Code/Node.js
- PHP, PHP 5.4 +.
- C#, .NET 4.5, Windows Store/Windows Phone, and Xamarin.
- C#, Unity.
- C, Arduino and other Embedded C Systems.
- Language Agnostic, REST API.
Pricing on Parse
Parse has a pricing structure that is pretty nice as you get started. You can get started for free and use up to 30 requests per-second until you reach a threshold to where you need to sign up for a paid plan. After that the pricing plans are a bit steep as you need more bandwidth and can increase at a rate of 100$ per month per 10 requests per-second. So, for example, if you need 40 requests per-second your costs are 100$ a month. If you need 50 requests per-second your costs are 200$ a month. The rates keep going up at a cost of 100$/10 request per-second and can be pro-rated based upon your plan. This may seem a bit steep as you pass the first couple of thresholds, but if you do the math on the total amount of bandwidth and the fact that you do not have to maintain the infrastructure, it becomes a pretty compelling pricing plan.
Analytics on Parse
Another great feature Parse has to offer is the Parse Analytics that are built right into the dashboard of your application. Without having to do anything Parse will track app installations, crashes, API requests, push notifications, and users. All of this data is presented then to you via Analytics tab on your apps dashboard through charts, graphs, and data tables. Custom analytics are available too as Parse has setup custom classes for tracking specific data about your application that you can send into the datastore just like you would if you were saving an object to the datastore. The best thing about Parse Analytics is that it is totally free to send an analytics request, it will not count against your request per-second.
Things to consider when getting involved with Parse
Although there are many things to love about Parse and how it can expedite the development process, there are a couple of things to keep in mind when planning to develop on Parse. Number one is if you go over the current rate plan you are on, Parse will start dropping requests to keep you within your limits. Auto-scaling is something that you need to be watching on your own to ensure that you can throttle your requests accordingly. Number two is if your application requires specific backup needs you will be responsible for the manual backup of the Parse data yourself. There is not an automatic task to snapshot your data specifically. For more information, here is a link to the May edition of Ask Parse Anything in which this topic was covered: https://youtu.be/7mmnW2p6c2k?t=794 . Number three, if your application requires a service level agreement (SLA), or an up-time contract, Parse will not provide this for you. Personally, I have never seen a service issue, but it should be noted.
Parse APIs
I have been involved with Parse projects on iOS, Android, PHP, client side JS, and server side JS via Cloud Code and so far I do not have a bad thing to say about any of their APIs or documentation. I think two areas where they really excel is number one, from the mobile side, syncing data behind the scenes with your remote Parse data store, and number two, making client side JavaScript applications in either Backbone JS or React JS. In respect to mobile platforms opening up your code and querying from either the local datastore or over the network without having to setup anything with your servers or your mobile application is an amazing time saver and extremely memory efficient. From a the web side, Parse's JavaScript library is built using a fork of Backbone.js so if you are taking a traditional MVC approach and are creating a web application, Parse gives you the tools to make this web app leaner and meaner without having to write the code necessary to sync up with server endpoints. For a moderately sized application, Parse is a pretty solid technical choice.